Why we need URL encoding?
May 02, 2021
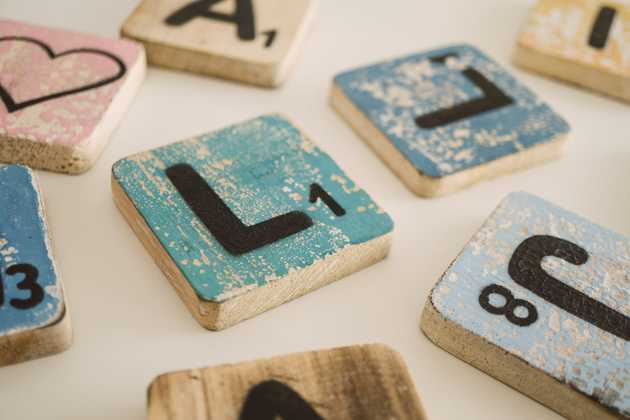
URL encoding converts unsafe characters into a valid ASCII character-set, which is a format that
can be transmitted over the Internet. The unsafe characters are e.g. non-latin letters and spaces.
These characters need to be replaced with their UTF-8 codes, e.g. space is replaced with %20
,
question mark is replaced with %3F
, @ is replaced with %40
etc.
URL encoding functions
To URL encode or decode the string you can use built-in javascript global functions:
- encodeURI() - we use it on a whole URL string, it encodes special characters except:
- _ . ! ~ * ' ( ) # ; , / ? : @ & = + $
- decodeURI() - decodes it back
- encodeURIComponent() - we use it on a part of the URL such as a query string / search
parameter, a hash, or a pathname, which are components of a URL, it encodes special characters
except:
- _ . ! ~ * ' ( )
- decodeURIComponent() - decodes it back
http://www.example.com/?q=hi%20there
We can use URL encoding while sending web form data
We need to encode not only a URL or a URL component. The user-entered fields sent from a web form to the server can also be URL encoded. There are three encoding types of forms (enctype attribute), but only the first one uses URL encoding:
application/x-www-form-urlencoded
- the default, data that is submitted using this type of form is URL encoded; you don’t actually need to write enctype in the<form>
element because it is the defaultmultipart/form-data
- when the user needs files to be uploaded to the server, data is not URL encodedtext/plain
- the newest type introduced in HTML5, sends the data without any encoding
application/x-www-form-urlencoded format
The data entered via a web form is submitted to the server using a POST request, in which body (i.e. data) is URL encoded. You can also see the content type in the request headers.
Content-Type: "application/x-www-form-urlencoded"
The data is encoded just like with encodeURIComponent() function but there is one difference. The
space is encoded as a +
sign and not %20
(for historical reasons).
email=hello%40example.pl&pass=pa%24%24word&feeling=I+fill+great
The URL encoded body looks very similar to query string parameters in a URL, both are transferred to
the server in this format: key1=value1&key2=value2
In Node.js when you want the incoming request data to be parsed you can use a built-in
middleware Express function express.urlencoded(), or a 3rd party body parsing middleware
bodyParser.urlencoded() in order to get access to the data in req.body
object in the format of
key: value
:
{
email: "hello@example.pl",
pass: "pa$$word",
feeling: "I fill great",
}
Then you can write the data to the database or process it the way you want.